Bitwise Operator in Java
In Java, an operator is a symbol that performs the specified operations. In this section, we will discuss only the bitwise operator and its types with proper examples.
Types of Bitwise Operator
There are six types of the bitwise operator in Java:
- Bitwise AND
- Bitwise exclusive OR
- Bitwise inclusive OR
- Bitwise Compliment
- Bit Shift Operators
Operators | Symbol | Uses |
---|---|---|
Bitwise AND | & | op1 & op2 |
Bitwise exclusive OR | ^ | op1 ^ op2 |
Bitwise inclusive OR | | | op1 | op2 |
Bitwise Compliment | ~ | ~ op |
Bitwise left shift | << | op1 << op2 |
Bitwise right shift | >> | op1 >> op2 |
Unsigned Right Shift Operator | >>> op >>> | number of places to shift |
Let's explain the bitwise operator in detail.
Bitwise AND (&)
It is a binary operator denoted by the symbol &. It returns 1 if and only if both bits are 1, else returns 0.
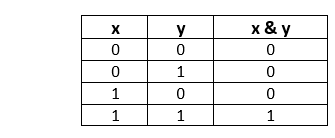
Let's use the bitwise AND operator in a Java program.
BitwiseAndExample.java
Output
x & y = 8
Bitwise exclusive OR (^)
It is a binary operator denoted by the symbol ^ (pronounced as caret). It returns 0 if both bits are the same, else returns 1.
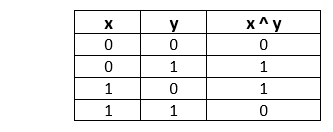
Let's use the bitwise exclusive OR operator in a Java program.
BitwiseXorExample.java
Output
x ^ y = 1